- In this section of python we will learn data types, variables, operators and expression in detail.
- Python has a predefined set of data types to handle the data in a program.
- We can store any type of data in python.
Data types
- Data can be of any type like- character, integer, real, string.
- Anything enclosed in ““is considered as string in Python.
- Any whole value is an integer value.
- Any value with fraction part is a real value.
- True or False value specifies Boolean value.
- Python has Two data types –
- Primitive Data Type (Numbers, String)
- Collection Data Type (List, Tuple, Set, Dictionary)
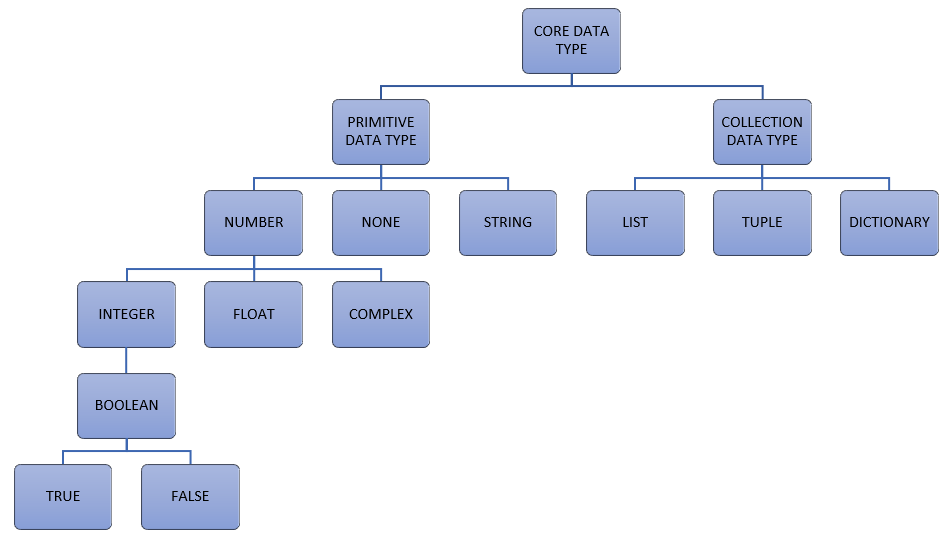
Numbers:
- Number data types store numeric values.
- There are three numeric types in python that are as follow:
- Integer
- Float
- Complex
INTEGER
- There are two types of integers in python are there:
- int
- Boolean
- Int:
- int or integer, is a whole number, positive or negative, without decimals.
- For example:
x = 1
y = 35656222554887711
z = -3255522
BOOLEAN
- It has two values: True and False. True has the value 1 and False has the value 0.
- For example:
>>>bool (0)
False
>>>bool (1)
True
>>>bool (‘‘)
False
>>>bool (-34)
True
>>>bool (34)
True
FLOAT
- float or “floating point number” is a number, positive or negative, containing one or more decimals. Float can also be scientific numbers with an “e” to indicate the power of 10.
- For example:
x = 1.10
y = 1.0
z = -35.59
a = 35e3
b = 12E4
c = -87.7e100
COMPLEX
- Complex numbers are written with a “i” as the imaginary part.
- Real and imaginary part of a number can be accessed through the attributes real and imag.
- For example:
>>>x = 3+5j
>>>y = 2+4j
>>>z= x + y
>>>print(z)
5+9j
>>>z. real
5.0
>>>z. imag
9.0
STRING
- Sequence of characters represented in the quotation marks.
- Python allows for either pairs of single or double quotes. Example: ‘hello’ is the same as “hello”.
- Python does not have a character data type; a single character is simply a string with a length of 1.
- The python string store Unicode characters.
- Each character in a string has its own index.
- String is immutable data type means it can never change its value in place.
NONE
- None represents a missing entry, but its type is not numeric. This means that any column (Series) that contains a None cannot be of type numeric (e.g., int and float).
LISTS
- A list is a data type that allows you to store various types data in it.
- It is a compound data type which means you can have different-2 data types under a list, for example we can have integer, float and string items in a same list.
- To create a list all you have to do is to place the items inside a square bracket [] separated by comma (,).
- Syntax to access the list items:
- list_name[index]
- For example:
num = [11.22, 9.9, 78.34, 12.0]
mix = [1.13, 2, 5, “book”, 100, “hi”]
TUPLE
- A tuple is an ordered sequence of elements of different data types, such as integer, float, string or list.
- Elements of a tuple are enclosed in parenthesis (round brackets) and are separated by commas.
- For example:
>>> a = (1, ‘a’, 7, 6.5) # a is the tuple of mixed data type
>>> b = (2, 4, 6, 8, 10) # b is a tuple of only integers
>>> c = (“English”, “Hindi”, “Math”, “Science”) # c is a tuple of only string
>>> d = (2, 4, 7, [4, 5, 6]) # d is a tuple with list as an element
DICTIONARY
- Dictionaries are Python’s implementation of a data structure that is more generally known as an associative array.
- A dictionary consists of a collection of key-value pairs. Each key-value pair maps the key to its associated value.
- You can define a dictionary by enclosing a comma-separated list of key-value pairs in curly braces ({}). A colon (:) separates each key from its associated value.
- Syntax for it is:
- d = {<key>: <value>, <key>: <value>, <key>: <value>, . . . .}
- For example:-
team = {'Colorado' : 'Rockies', 'Boston' : 'Red Sox', 'Minnesota': 'Twins', 'Milwaukee': 'Brewers', 'Seattle' : 'Mariners' }
SET
- It is an unordered collection of unique and immutable (which cannot be modified) items.
- For example:-
set1={11,22,33,22}
print(set1)
- output
{33, 11, 22}
EXPRESSIONS
- An expression in python is any valid combination operators, literals and variables.
- An expression is python is any valid combination of operation and atoms. As expression are the composed of one or more operation.
ATOMS
- An atom is something that has a value.
- Identifiers, literals, string, lists, tuples, sets, dictionaries, etc. are all atoms.
Python offers 4 types of expressions that as follow
ARITHMETIC EXPRESSION
- These expressions involve numbers (integers, floating-point numbers, complex numbers) and arithmetic operators.
RELATIONAL EXPRESSION
- An expression having literals and/or variables of any valid type and relational operators is a relational expression.
LOGICAL EXPRESSION
- An expression having literals and/or variables of any valid type and logical operators is a logical expression.
STRING EXPRESSION
- Python also provides two string operators + and *, when combined with string operands and integers, form string expressions.
- With operator +, the concatenation operator, the operands should be one string type only.
- With * operator, the replication operator, the operands should be one integer.
NOTE : –
Learn Python Programming from Basics– Click here
Installation Process of Python– Click here
5 Tips to Learn python Faster – Click here